Algorithms and data structures are fundamental concepts in the field of computer science. They provide the foundation for solving complex problems and effectively processing large amounts of data. From creating efficient search engines to building intelligent systems, algorithms and data structures play a crucial role in the development of software and technology.
In this article, we will explore the basics of algorithms and data structures, their importance, common types, and real-world applications. By the end, you will have a better understanding of these essential concepts and how they apply to various fields in computer science.
Importance of Algorithms and Data Structures
Before diving into the details, let’s first understand why algorithms and data structures are so important. The main reason is that they help us solve problems efficiently. In today’s world, where data is constantly growing and new challenges arise every day, having the ability to process and analyze information quickly is essential.
Without proper algorithms and data structures, it would be nearly impossible to handle the vast amount of data generated by modern technology. For example, consider search engines like Google, which process millions of search queries every second. Without efficient algorithms and data structures, it would take too long to deliver results and make the user experience frustrating.
Moreover, algorithms and data structures also play a significant role in optimizing the performance of computer programs. By choosing the right algorithm and data structure for a specific task, developers can ensure that their software runs smoothly and efficiently. This not only enhances the user experience but also reduces the load on hardware resources, ultimately saving time and money.
Basic Concepts of Algorithms
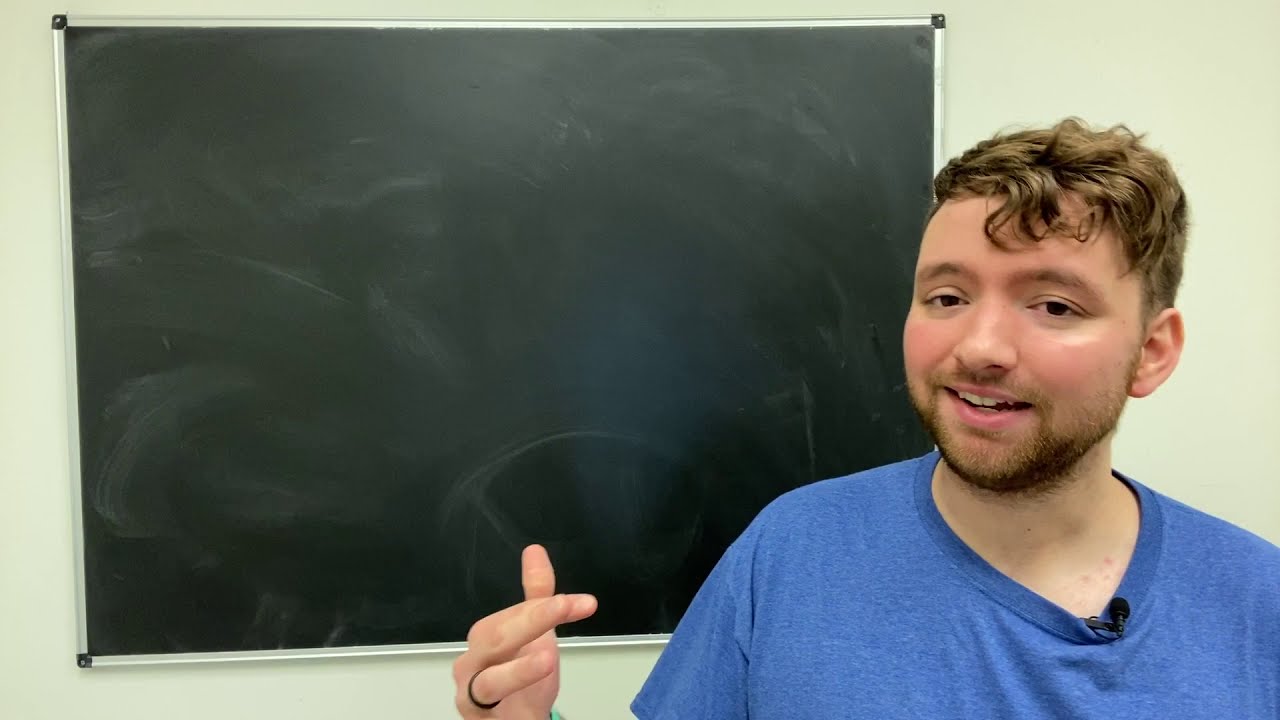
An algorithm is a step-by-step procedure used to solve a problem or perform a specific task. It takes an input, performs a series of operations, and produces an output. In computer science, algorithms are designed to process and manipulate data, making them an essential component in software development.
Characteristics of Algorithms

There are several characteristics that define an algorithm and make it different from a simple set of instructions. These include:
- Finiteness: An algorithm must have a finite number of steps to reach the desired result.
- Definiteness: Each step in an algorithm must be precisely defined and unambiguous.
- Input: Algorithms require some form of input data to perform their operations.
- Output: After executing all steps, an algorithm must produce a result or output.
- Effectiveness: An algorithm should be effective, meaning it should solve the problem using a reasonable amount of time and resources.
- Generality: An algorithm should be general enough to solve a range of similar problems.
Analysis of Algorithms
One crucial aspect of algorithms is analyzing their performance. This involves measuring how much time and space an algorithm requires to execute for a given input size. By analyzing the time and space complexity, we can determine the efficiency and scalability of an algorithm.
The time complexity of an algorithm refers to the number of operations it takes to complete for a given input size. It is typically measured in terms of Big O notation, which expresses the worst-case scenario for the algorithm’s performance. The lower the time complexity, the more efficient the algorithm is.
On the other hand, the space complexity of an algorithm measures the amount of memory required to execute the algorithm for a given input size. It is also expressed in Big O notation, with a lower space complexity indicating that the algorithm uses less memory and is more efficient.
Types of Algorithms
There are various types of algorithms depending on their purpose and functionality. Some common types include:
- Sorting Algorithms: These algorithms arrange a list of elements in a specific order, such as ascending or descending. Examples include bubble sort, quicksort, and merge sort.
- Searching Algorithms: These algorithms look for a specific element within a collection of data. Binary search and linear search are two common examples.
- Graph Algorithms: These algorithms are used to solve problems related to graphs, such as finding the shortest path between two nodes. Some common graph algorithms include Dijkstra’s algorithm and Prim’s algorithm.
- Greedy Algorithms: These algorithms make locally optimal choices at each step to find the overall optimal solution. The knapsack problem is an example of a problem solved using a greedy algorithm.
- Dynamic Programming Algorithms: These algorithms use a divide and conquer approach to break down a large problem into smaller subproblems and solve them recursively. Examples include the knapsack problem and the Fibonacci sequence.
Basic Concepts of Data Structures
Data structures are ways of organizing and storing data in a computer to facilitate efficient access and modification. They provide a logical representation of data and allow for easy manipulation of information. Just like algorithms, data structures are also essential in software development, as they help optimize the performance of computer programs.
Characteristics of Data Structures
There are several characteristics that define a data structure and make it suitable for different scenarios. These include:
- Efficiency: A data structure should be efficient in terms of time and space complexity.
- Ease of use: It should be easy to understand and manipulate the data stored within a data structure.
- Scalability: A good data structure should work efficiently for different sizes of data inputs.
- Flexibility: Data structures should be flexible enough to handle various types of data and operations.
- Modularity: Data structures should be modular, meaning they can be combined with other data structures to perform more complex operations.
Types of Data Structures
There are many different types of data structures, each designed for specific purposes and scenarios. Some common ones include:
- Arrays: An array is a collection of elements stored in contiguous memory locations. It allows for random access and is useful for operations that require frequent indexing, such as sorting and searching algorithms.
- Linked Lists: A linked list is a linear data structure, where each element contains a reference to the next element in the list. It is useful for implementing stacks, queues, and other data structures.
- Stacks: A stack is a data structure that follows the Last In, First Out (LIFO) principle. It allows you to add elements at the top and remove them from the top only.
- Queues: A queue is a data structure that follows the First In, First Out (FIFO) principle. It allows you to add elements at the end and remove them from the front only.
- Trees: A tree is a hierarchical data structure with a root node and branches connecting to child nodes. It is useful for storing data in a sorted manner and performing operations like searching and sorting.
- Graphs: A graph is a non-linear data structure consisting of nodes connected by edges. It is useful for modeling relationships between objects and solving problems related to networks and connectivity.
- Hash Tables: A hash table is a data structure that stores key-value pairs in a way that makes it easy to retrieve the value corresponding to a given key. It is useful for fast lookup operations and is commonly used in database systems.
Common Algorithms and Data Structures
Now that we have covered the basics of algorithms and data structures let’s explore some common examples that you are likely to encounter in your computer science journey.
Sorting Algorithms
Sorting algorithms are used to organize a list of elements in a specific order. They are an essential part of many real-world applications, such as organizing data in databases, finding the most relevant search results, and displaying items in order on an e-commerce website.
One of the most well-known sorting algorithms is the bubble sort algorithm. It works by comparing adjacent elements and swapping them if they are in the wrong order. The process repeats until the list is sorted. While bubble sort is simple to understand, it has a time complexity of O(n^2), making it less efficient for large data sets.
Another popular sorting algorithm is quicksort, which follows a divide and conquer approach. It works by selecting a pivot element and dividing the list into two sublists – one with elements smaller than the pivot and another with elements larger than the pivot. The process repeats recursively until the entire list is sorted. Quicksort has an average time complexity of O(n log n) but can deteriorate to O(n^2) in worst-case scenarios.
Searching Algorithms
Searching algorithms are used to find a specific element within a collection of data. They are used in many real-world applications, such as searching for a file on your computer or finding a particular record in a database.
One of the most common searching algorithms is linear search, where the algorithm iterates through each element in a list until it finds the desired element. This algorithm has a time complexity of O(n), where n is the number of elements in the list.
Another popular searching algorithm is binary search, which can only be applied to a sorted list. It works by repeatedly dividing the list in half and checking if the desired element is in the higher or lower half. This process continues until the element is found or the list is exhausted. Binary search has a time complexity of O(log n), making it more efficient for larger data sets compared to linear search.
Trees
Trees are hierarchical data structures that consist of nodes connected by edges. They are used to represent relationships between objects and efficiently store and retrieve data. There are several types of trees, including binary trees, binary search trees, and balanced trees.
A binary tree is a tree where each node can have at most two child nodes – a left child and a right child. Binary trees are often used to implement other data structures like binary heaps and binary search trees. They have a time complexity of O(n) for most operations, including searching and insertion.
Binary search trees (BSTs) are special types of binary trees that are commonly used in search algorithms. They follow a specific ordering principle, where all elements in the left subtree of a node are smaller than the node, and all elements in the right subtree are larger. This makes it easier to search for an element in a BST, as you can eliminate half the nodes at each step, resulting in a time complexity of O(log n).
Graph Algorithms
Graphs are non-linear data structures consisting of nodes and edges. They are used to represent relationships between objects and solve problems related to connectivity and networks. Some common graph algorithms include Dijkstra’s algorithm and Prim’s algorithm.
Dijkstra’s algorithm is used to find the shortest path between two nodes in a graph. It works by calculating the shortest distance from the starting node to all other nodes in the graph. This algorithm has a time complexity of O(E + V log V), where E is the number of edges and V is the number of vertices in the graph.
Prim’s algorithm is used to find the minimum spanning tree of a weighted graph. A minimum spanning tree is a subset of edges that connect all vertices in a graph with the minimum total weight. Prim’s algorithm works by adding the shortest edge that connects a visited vertex to an unvisited vertex until all vertices are connected. It has a time complexity of O(E + V log V).
Applications of Algorithms and Data Structures
Algorithms and data structures are widely used in various fields of computer science and beyond. Let’s take a look at some real-world applications of these concepts.
Artificial Intelligence and Machine Learning
Artificial intelligence (AI) and machine learning (ML) are two rapidly growing fields that rely heavily on algorithms and data structures. AI algorithms, such as neural networks, use complex mathematical models to learn from data and perform tasks that typically require human intelligence. These algorithms require efficient data structures, such as arrays and matrices, to store and manipulate the vast amounts of data they process.
Machine learning algorithms also heavily rely on data structures to store and organize training data. For example, decision tree algorithms use binary trees to represent rules for making decisions based on input data. Support vector machines (SVMs) use hash tables to store support vectors, which are essential in classifying new data points.
Search Engines
Search engines use a variety of algorithms and data structures to provide users with relevant search results quickly. For example, when you enter a search query on Google, the engine uses an algorithm called PageRank to rank pages based on their relevance and authority. This algorithm relies on various data structures, such as graphs and hash tables, to process the vast amounts of data generated by the Internet.
Search engines also use algorithms such as crawling and indexing to gather and organize information from the web. These algorithms require efficient data structures, such as trees and queues, to store and retrieve data efficiently.
Database Management Systems
Database management systems (DBMS) use algorithms and data structures to store, organize, and retrieve large amounts of data. Some common data structures used in DBMS include arrays, linked lists, and trees. These data structures allow for fast lookup operations and efficient handling of data.
DBMS also use algorithms like sorting and searching to optimize database queries and make data retrieval more efficient. These algorithms require proper data structures, such as B-trees, to ensure quick access to the desired data.
Gaming and Graphics
Algorithms and data structures are crucial in game development and computer graphics. In games, algorithms are used to determine the behavior of non-player characters (NPCs), generate random elements, and simulate physics. Data structures like arrays and linked lists are used to store game objects and keep track of their properties.
In graphics, algorithms are used to create realistic 3D models and animations. Data structures like matrices and graphs are used to store the coordinates and relationships between different points in a 3D space. Rendering algorithms then use this data to create a visual representation of the scene.
Conclusion
In conclusion, algorithms and data structures are essential concepts in computer science that play a crucial role in solving complex problems efficiently. They provide the foundation for software development and are widely used in various fields such as artificial intelligence, search engines, and gaming.
In this article, we explored the basics of algorithms and data structures, their characteristics, types, and real-world applications. Understanding these concepts is essential for anyone pursuing a career in computer science and will help you become a better problem solver and software developer. So keep learning and exploring, and remember that with the right algorithms and data structures, anything is possible!