Robot Operating System (ROS) is a popular open-source framework for robot programming. It provides a collection of tools, libraries, and conventions for building complex robotic systems. With its modular and distributed architecture, ROS enables developers to easily integrate different hardware components and control them using high-level programming languages. In this blog post, we will explore the basics of programming robots with ROS, from setting up the environment to advanced topics in ROS programming.
Introduction to ROS
ROS was initially developed in 2007 by Willow Garage, an American robotics research lab, as a way to simplify the development of personal robots. Since then, it has been widely adopted by both academic and industrial communities and has become the de facto standard for robot programming.
One of the key features of ROS is its flexibility and modularity. It allows for easy integration of different modules or nodes, which can communicate with each other over a network. This distributed architecture makes it possible to build complex robotic systems that are not limited to a single machine. Additionally, ROS supports multiple programming languages such as C++, Python, and Java, making it accessible to a wider community of developers.
Another important aspect of ROS is its active and supportive community. The codebase is constantly being updated and improved, and there are numerous online resources and forums where developers can seek help and share their work. This collaborative approach has led to the development of a vast library of packages and tools, making ROS one of the most versatile frameworks for robot programming.
Basics of programming robots
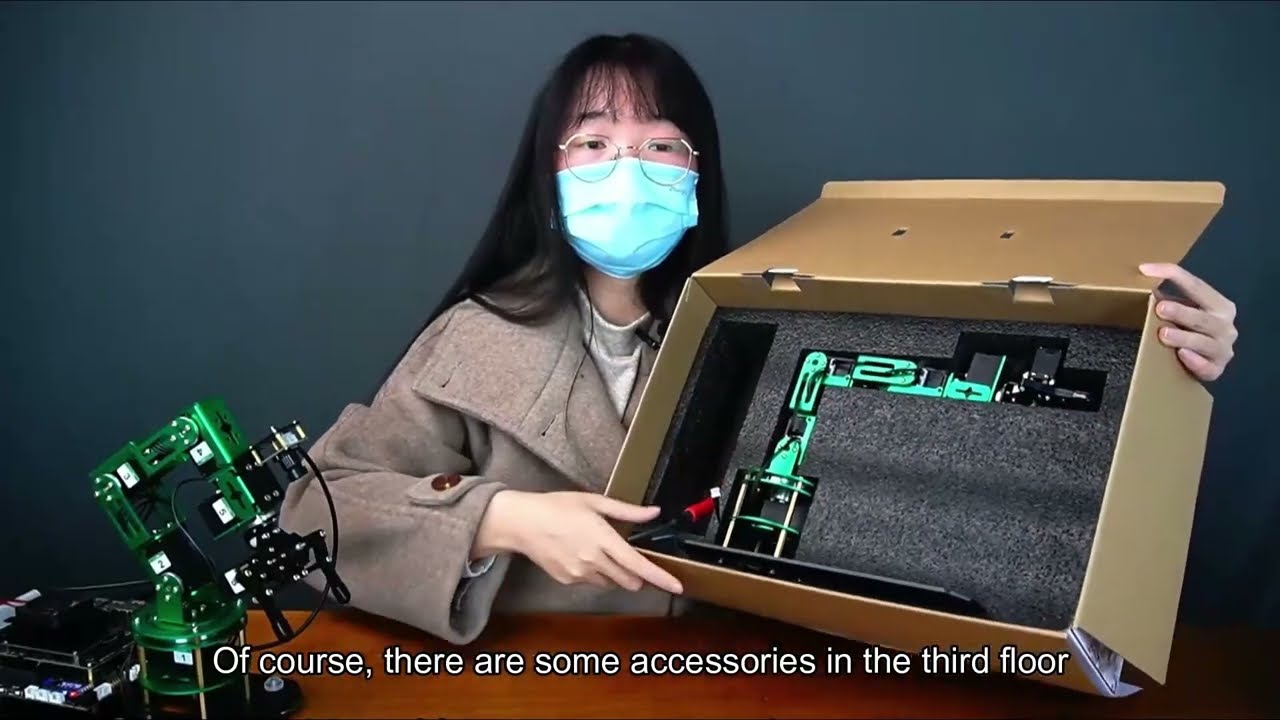
Before diving into the specifics of ROS programming, it is essential to understand the basics of robot programming as a whole. Robot programming involves writing code to control the behavior and movements of a robot, either manually or autonomously. There are various levels of programming involved in robot programming, from low-level microcontroller programming to high-level AI algorithms.
The first step in robot programming is to understand the hardware components of a robot, such as sensors, actuators, and controllers. These components are responsible for gathering data and interacting with the environment, which is then processed by the software. Next, programmers need to determine the desired behavior or task that the robot should perform. This could be something as simple as moving from one point to another or as complex as navigating an unknown environment.
Once the hardware and behavior are defined, the next step is to select the appropriate programming language and framework. As mentioned earlier, ROS supports multiple languages, but it is primarily used with C++ and Python. The choice of language depends on the programmer’s preference and the requirements of the project.
Overview of ROS architecture

ROS follows a distributed architecture, where different nodes communicate with each other over a network. It consists of three main components: the Master, Nodes, and Messages.
The Master
The Master is the central node in ROS that manages communication between different nodes. It maintains a registry of all active nodes, their published and subscribed topics, and the messages being exchanged. All nodes must register with the Master before they can communicate with each other.
Nodes
Nodes are standalone processes that perform specific tasks and communicate with each other through the Master. Each node can have multiple publishers and subscribers, allowing for data exchange between different nodes. For example, one node may control the movements of a robot, while another node may process sensor data and send it to the first node. This modular approach makes it easy to add or remove nodes without affecting the overall system.
Messages
Messages are the unit of data exchange in ROS. They are structured data types that contain information such as sensor readings, control commands, or any other data relevant to the robot’s functioning. Messages are defined in ROS using the Interface Description Language (IDL) and can be of different types, including strings, integers, arrays, and custom data structures.
Setting up ROS environment
Before jumping into programming robots with ROS, we need to set up our environment. The following steps will guide you through the installation process on a Linux machine.
- Install Ubuntu: ROS supports multiple versions of Ubuntu, but it is recommended to use the latest Long Term Support (LTS) release. As of now, the latest LTS release is Ubuntu 20.04.
- Install ROS: Once Ubuntu is installed, the next step is to install ROS. There are two main distributions of ROS: ROS 1 and ROS 2. In this blog post, we will focus on ROS 1, which is more widely used. Follow the instructions on the official ROS website to install the Melodic Morenia distribution.
- Set up the workspace: A workspace is a folder where you will store all your ROS-related projects and packages. Create a new folder for your workspace using the
mkdir
command. Next, navigate to thesrc
directory within your workspace and create a new package using thecatkin_create_pkg
command. This package will contain all the nodes and messages related to your project.
- Build the package: Once the package is created, navigate back to the root directory of your workspace and run the
catkin_make
command. This will build your package and its dependencies.
- Source the setup file: After the build process is complete, source the
setup.bash
file in your workspace’sdevel
directory using thesource
command. This will ensure that all ROS commands and packages are accessible from your current terminal session.
With these steps, your ROS environment is set up, and you are ready to start programming robots using ROS.
Programming robots using ROS
Now that we have a basic understanding of ROS and have our environment set up, let’s take a look at how we can program robots using ROS. In this section, we will go through the process of creating a simple robot control program using ROS.
Creating a publisher node
The first step in our programming journey is to create a publisher node that will send control commands to the robot. Create a new file called publisher_node.cpp
within the src
directory of your package and add the following code:
# include
# include
int main(int argc, char** argv) {
ros::init(argc, argv, "publisher_node");
ros::NodeHandle nh;
// Define the publisher
ros::Publisher pub = nh.advertise("control_commands", 1000);
ros::Rate loop_rate(10);
while(ros::ok()) {
// Create a message object
std_msgs::String msg;
// Populate the message
msg.data = "Move forward";
// Publish the message
pub.publish(msg);
// Sleep for 1 second
loop_rate.sleep();
}
return 0;
}
Let’s break down this code. First, we include the necessary header files for ROS and the message type we want to use. Then, we initialize the NodeHandle
object, which allows us to communicate with the Master and other nodes. Next, we define a publisher object, which is associated with the control_commands
topic and has a buffer size of 1000 messages.
In the while
loop, we create a message object of type std_msgs::String
, populate it with the desired control command, and publish it on the control_commands
topic. Finally, we use the sleep()
function to pause the loop for 1 second, ensuring that the command is not published too frequently.
Creating a subscriber node
Next, we need to create a subscriber node that will receive the control commands and act on them. Create a new file named subscriber_node.cpp
within the src
directory and add the following code:
# include
# include
void commandCallback(const std_msgs::String::ConstPtr& msg) {
// Print the received command
ROS_INFO("Received command: %s", msg->data.c_str());
}
int main(int argc, char** argv) {
ros::init(argc, argv, "subscriber_node");
ros::NodeHandle nh;
// Define the subscriber
ros::Subscriber sub = nh.subscribe("control_commands", 1000, commandCallback);
// Spin until the subscriber is shut down
ros::spin();
return 0;
}
Here, we include the necessary header files and define a callback function that will be called every time a message is received on the control_commands
topic. Inside the main()
function, we initialize the node, define a subscriber object, and specify the topic and buffer size. Finally, we call the spin()
function, which keeps the subscriber active until it is explicitly shut down.
Building and running the nodes
Now that our publisher and subscriber nodes are created, we need to build them and run them. Navigate back to the root directory of your workspace and run the catkin_make
command to build the package. Then, source the setup file using the source
command.
Next, open two terminal windows and navigate to the root directory of your workspace in both. In the first terminal, run the roscore
command to start the Master. In the second terminal, run the rosrun
command to execute the publisher and subscriber nodes:
$ rosrun publisher_node
$ rosrun subscriber_node
You should now see the control command being printed in the terminal where the subscriber node is running.
Adding a controller
Now, let’s take our program one step further by adding a controller that will act on the received control commands. Create a new file called controller.cpp
within the src
directory and add the following code:
# include
# include
void commandCallback(const std_msgs::String::ConstPtr& msg) {
// Get the received command
std::string command = msg->data;
// Check the command and perform appropriate action
if(command == "Move forward") {
ROS_INFO("Moving forward...");
// Code to move the robot forward
}
else if(command == "Move backward") {
ROS_INFO("Moving backward...");
// Code to move the robot backward
}
else if(command == "Turn left") {
ROS_INFO("Turning left...");
// Code to turn the robot left
}
else if(command == "Turn right") {
ROS_INFO("Turning right...");
// Code to turn the robot right
}
}
int main(int argc, char** argv) {
ros::init(argc, argv, "controller");
ros::NodeHandle nh;
// Define the subscriber
ros::Subscriber sub = nh.subscribe("control_commands", 1000, commandCallback);
// Spin until the subscriber is shut down
ros::spin();
return 0;
}
Similar to the subscriber node, we define a callback function to receive the control commands. Then, depending on the received command, we perform the appropriate action. For simplicity, we have only included four basic commands, but you can extend this program to include more complex actions and movements for your robot.
Build the package again using the catkin_make
command, and run the controller node using the rosrun
command.
$ rosrun controller
Now, when you run the publisher and subscriber nodes again in separate terminals, you should see the appropriate messages being printed in the terminal where the controller is running.
Congratulations! You have successfully programmed your first robot using ROS.
Advanced topics in ROS programming
As you gain more experience with ROS, you can explore some of its advanced features and tools that can enhance your robot programming skills.
Using the ROS development studio
The ROS Development Studio (ROSDS) is a browser-based platform that provides a virtual environment for developing and testing ROS projects. It comes pre-installed with all the necessary packages and libraries, making it ideal for beginners who want to get started with ROS without having to go through the installation process. Additionally, ROSDS provides tutorials and learning resources to help users get familiar with ROS and its concepts.
Simulating robots with Gazebo
Gazebo is a popular physics engine used for simulating robots and environments. It is integrated with ROS and allows developers to test their robot programs in a realistic virtual environment. With Gazebo, you can simulate sensors, actuators, and even entire robots, making it an invaluable tool for debugging and testing your code before deploying it on a physical robot.
Using RViz for visualization
RViz is a 3D visualization tool that comes bundled with ROS. It allows developers to visualize data from various sources, such as sensors, cameras, and robots, in a 3D environment. This is especially useful for debugging and understanding the behavior of your robot. RViz also supports plugins, making it highly customizable and extensible.
Implementing AI algorithms with ROS
ROS provides numerous libraries and tools for implementing artificial intelligence (AI) algorithms such as machine learning and computer vision. These can be used to enhance the capabilities of your robot, such as object recognition and navigation in complex environments. Additionally, ROS also has a dedicated library for building and training robots using reinforcement learning.
Conclusion and future prospects
In this blog post, we explored the basics of programming robots with ROS. We learned about its architecture, set up the environment, and created a simple program to control a robot. We also discussed some advanced features and tools that can be used to enhance our robot programming skills. With its widespread adoption and active community, ROS is expected to continue evolving and playing a significant role in the development of future robots. So, whether you are a beginner or an experienced developer, there is always something new to learn and explore in the world of ROS.