Data structures are an essential aspect of computer science and programming, and they play a crucial role in organizing and managing data efficiently. In simple terms, data structures are the different ways of organizing and storing data in a computer’s memory. They provide a systematic approach to storing and retrieving data, making it easier for programmers to develop efficient algorithms and programs.
In this blog post, we will explore the concept of data structures, their importance, types, basic operations, common data structures used in programming, and their applications. This comprehensive guide will serve as a foundation for understanding data structures and their role in software development.
Definition of Data Structures
Before delving into the details, let’s first define what data structures are. As mentioned earlier, data structures are the different ways of organizing and storing data in a computer’s memory. They can be thought of as containers that hold data in a structured manner, making it easier to retrieve and manipulate.
Think of a file cabinet in an office – it has drawers, folders, and files, all organized in a specific way to store and access information efficiently. Similarly, data structures organize data in a computer’s memory, allowing programmers to perform operations on them effectively.
Importance of Data Structures
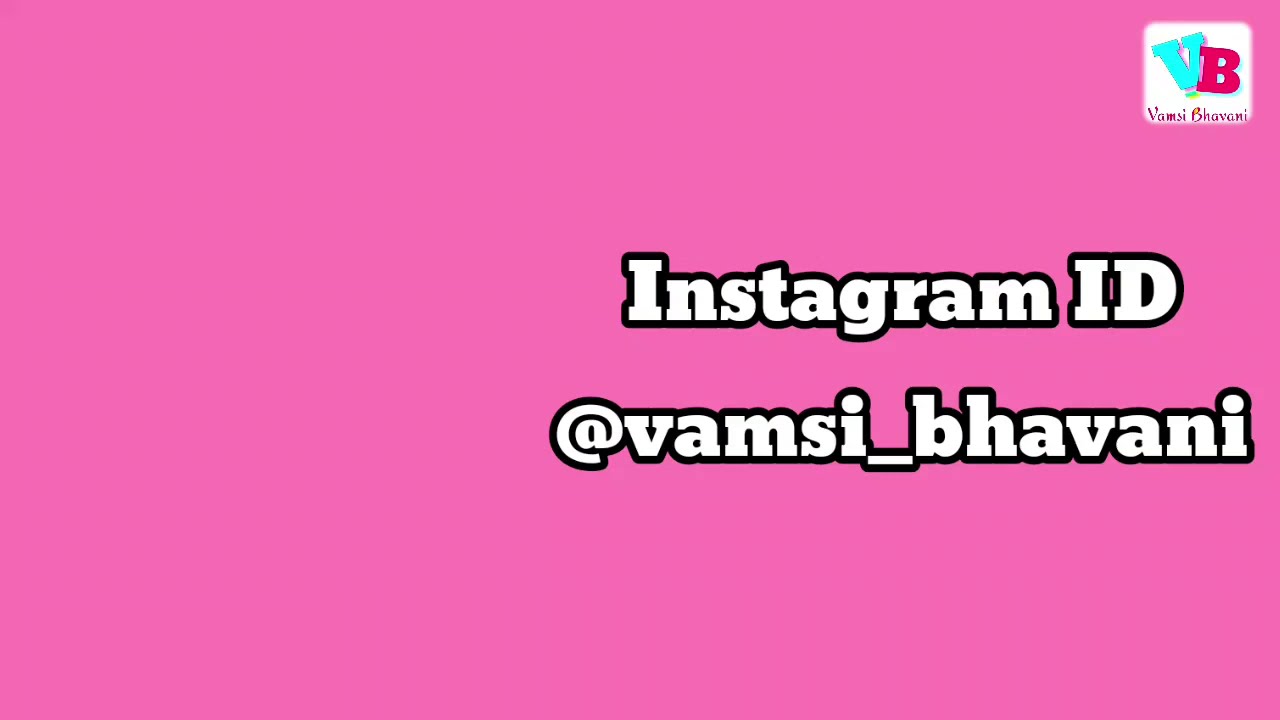
Data structures are crucial in computer science and programming for the following reasons:
Efficient Data Storage and Retrieval
Efficient data storage and retrieval are the key benefits of using data structures. When data is organized and stored in a structured manner, it becomes easier to search, insert, and delete. This leads to better performance and faster execution of programs, making data structures essential in developing high-performing software.
Better Memory Management
Another crucial aspect of data structures is memory management. With proper data structures, memory usage can be optimized, leading to the efficient utilization of resources. This is especially important in large-scale applications where memory usage needs to be optimized for better performance.
Better Understanding of Algorithms and Programming
Data structures are the building blocks of algorithms and programming languages. By understanding different data structures, programmers can better understand how algorithms work and develop efficient solutions to various problems. It also helps in understanding the advantages and disadvantages of different data structures and choosing the right one for a particular problem.
Types of Data Structures
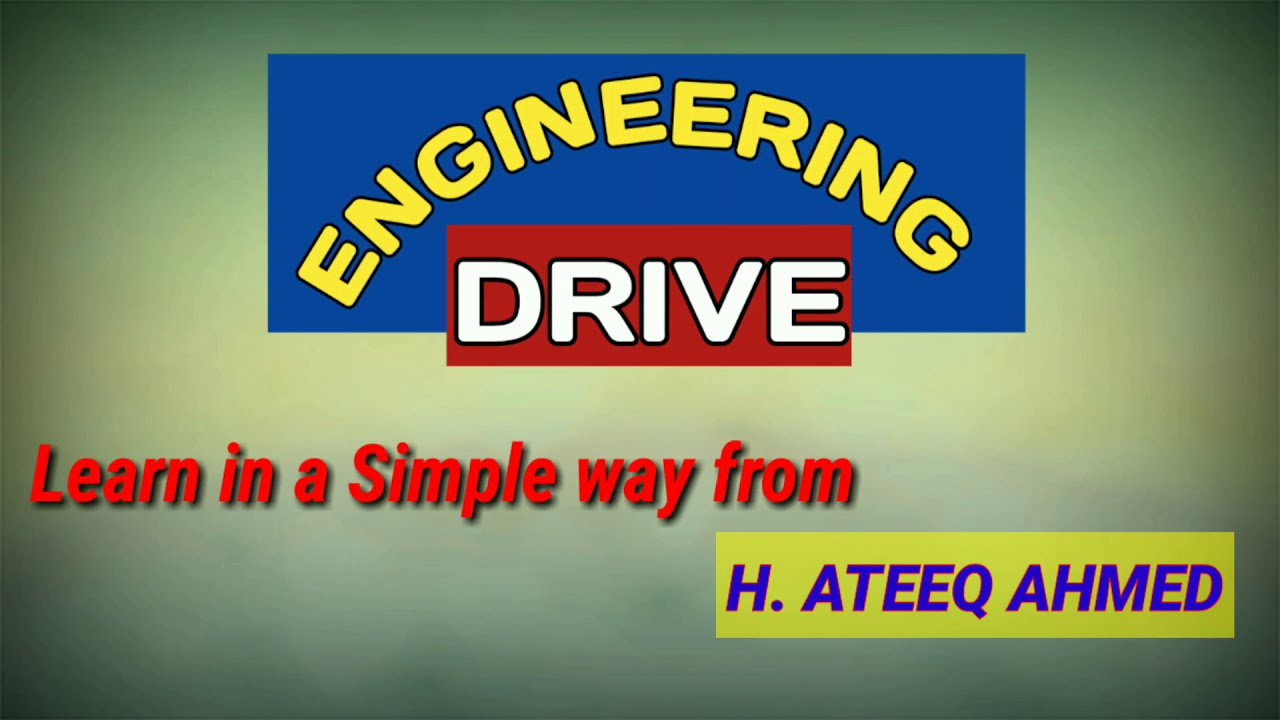
There are various types of data structures, each with its unique characteristics and uses. Here are some of the most commonly used data structures:
Array
An array is a linear data structure that stores a collection of elements of the same type. It has a fixed size, and the elements are stored in contiguous memory locations. The main advantage of arrays is their fast access time, making them ideal for applications where fast retrieval of data is essential. However, arrays have a fixed size, which can be a disadvantage if the number of elements exceeds the array’s capacity.
Linked List
A linked list is another linear data structure that consists of nodes connected together via pointers. Unlike arrays, linked lists do not have a fixed size and can dynamically adjust to accommodate new elements. This makes it more flexible than arrays but also leads to slower access times. Linked lists are commonly used in data structures like stacks, queues, and graphs.
Stack
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle, meaning the last element added to the stack will be the first one to be removed. It can be implemented using arrays or linked lists and supports operations such as push (to add an element) and pop (to remove an element). Stacks are commonly used in applications that require backtracking, such as web browsers and compilers.
Queue
A queue is a linear data structure that follows the First In, First Out (FIFO) principle, meaning the first element added to the queue will be the first one to be removed. It can also be implemented using either arrays or linked lists and supports operations such as enqueue (to add an element) and dequeue (to remove an element). Queues are commonly used in applications that require a waiting line, such as operating systems and computer networks.
Tree
A tree is a non-linear data structure that consists of nodes connected together in a hierarchical manner. It has a root node at the top, followed by various child nodes branching out from it. Trees are used to represent hierarchical structures like family trees, organization charts, and file systems. They are also used in search algorithms like binary search trees and in data compression techniques like Huffman coding.
Hash Table
A hash table is a data structure that uses a hashing function to map keys to values. It allows for fast insertion, deletion, and retrieval of data, making it ideal for applications that require quick access to data. However, if the number of elements exceeds the hash table’s capacity, it can lead to collisions, which can affect its performance.
Basic Operations on Data Structures
Data structures support various operations that allow programmers to manipulate the data stored in them efficiently. Here are some of the common operations performed on data structures:
Traversal
Traversal refers to accessing all the elements in a data structure one by one. It is an essential operation as it allows programmers to perform other operations on the data, such as searching, sorting, and inserting.
Searching
Searching is the process of finding a particular element in a data structure. The efficiency of searching depends on the type of data structure used. For example, searching in a sorted array is faster compared to searching in an unsorted array.
Sorting
Sorting is the process of arranging the elements in a data structure in a specific order, usually ascending or descending. There are various sorting algorithms, each with its advantages and disadvantages, depending on the type of data structure used.
Insertion and Deletion
Insertion involves adding new elements to a data structure, while deletion refers to removing existing elements from it. Both these operations are crucial in managing data and maintaining the integrity of the data structure.
Common Data Structures used in Programming
Different programming languages have built-in data structures that allow programmers to store and manage data efficiently. Here are some of the commonly used data structures in programming:
C++
C++ has a wide range of data structures, including arrays, vectors, queues, stacks, sets, and maps. It also supports user-defined data structures like structures and classes, making it a powerful language for developing complex software.
Java
Java has an extensive collection of data structures in its standard library, such as ArrayList, LinkedList, HashSet, and HashMap. It also supports user-defined data structures through classes and interfaces, making Java a popular choice for building large-scale applications.
Python
Python has built-in data structures such as lists, tuples, dictionaries, and sets. It also supports third-party libraries like NumPy and Pandas, which offer more advanced data structures for scientific computing and data analysis.
JavaScript
JavaScript has a relatively small set of built-in data structures, including arrays, objects, and maps. However, it has a vast collection of libraries and frameworks, such as React and Angular, that provide more advanced data structures and algorithms for web development.
Applications of Data Structures
Data structures have numerous applications in various fields, including computer science, engineering, finance, and healthcare. Here are some of the common applications of data structures:
Databases
Databases use data structures to store and retrieve large amounts of data efficiently. They allow for quick searching, sorting, and indexing of data, making them essential for applications that require fast retrieval of information, such as banking systems and e-commerce websites.
Operating Systems
Operating systems use data structures like queues and stacks to manage processes and resources efficiently. For example, the CPU scheduling algorithm uses a queue to manage multiple processes, while the undo/redo feature in text editors uses a stack to keep track of the changes made to a document.
Artificial Intelligence and Machine Learning
Data structures play a crucial role in artificial intelligence and machine learning algorithms. They are used to store and manipulate large datasets and perform operations like sorting, searching, and inserting in real-time, making them essential for developing intelligent systems.
Web Development
Web developers use data structures to manage and display data on websites. For example, arrays are used to store and display items in a shopping cart, while linked lists are used to create dynamic menus and navigation bars.
Conclusion
In conclusion, data structures are an essential aspect of computer science and programming. They provide a systematic way of organizing and managing data, making it easier for programmers to develop efficient algorithms and programs. In this blog post, we have explored the definition, importance, types, basic operations, common data structures used in programming, and applications of data structures. Understanding data structures is crucial for any aspiring programmer, as it forms the foundation for learning more complex concepts in computer science and software development.